- Published on
✨ React JS 초보자를 위한 리액트 강좌 #14 강의 정리
- 글쓴이
📌 목차
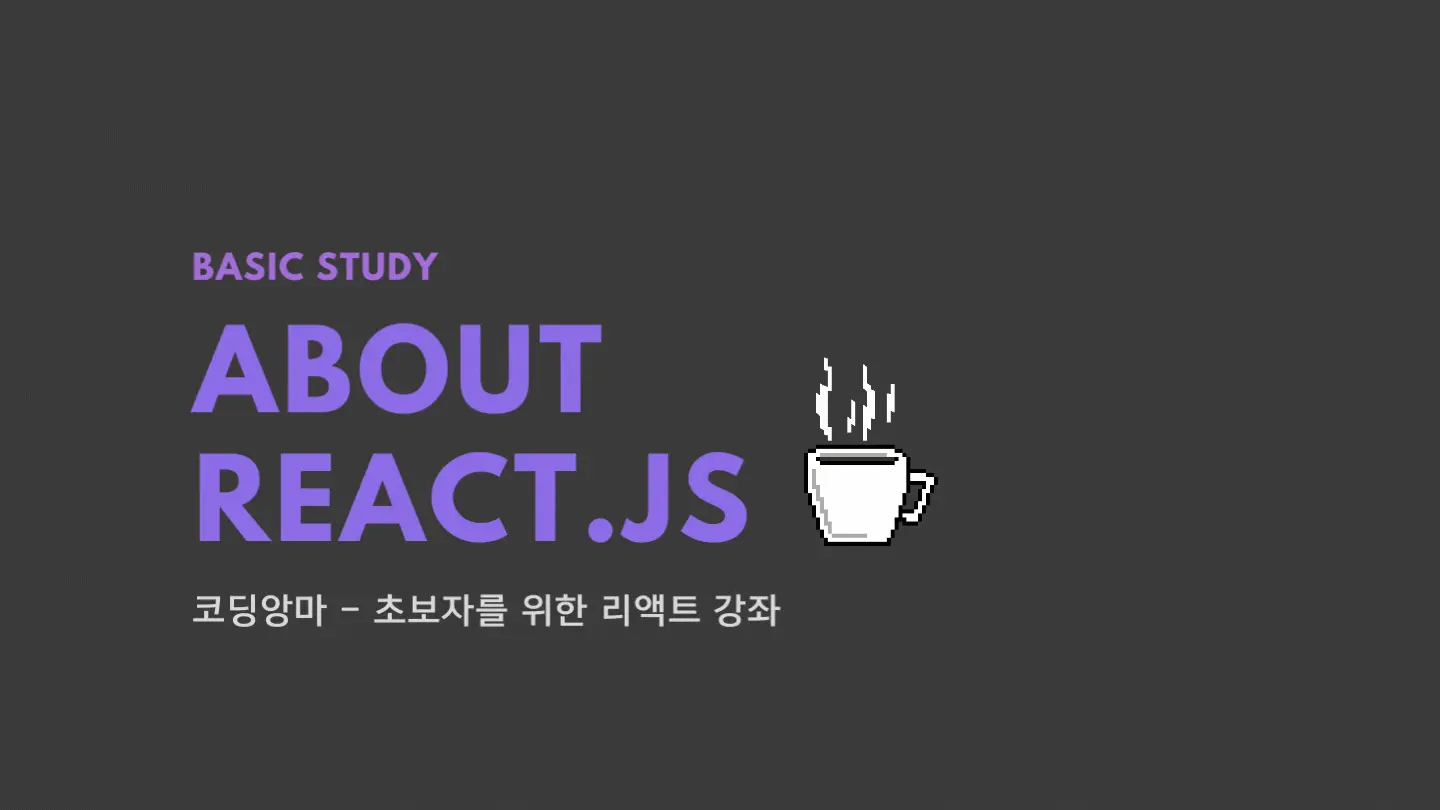
💁🏻
- 강의 소개
- 설치(create-react-app)
- 컴포넌트,JSX
- 첫 컴포넌트 만들기
- CSS 작성법
- 이벤트 처리
- state,useState
- props
- 더미 데이터 구현
- 라우터 구현 react-router-dom
- json-server,REST API
- useEffect,fetch()로 API 호출
- custom Hooks
- PUT(수정),DELETE(삭제)
- POST(생성),useHistory
- 마치며
- 부록: 타입스크립트 적용하기
PUT(수정),DELETE(삭제)
각 함수를 만들고 fetch
와 then
을 이용해서 코드를 작성한다.
import { useState } from "react";
export default function Word({ word: w }) {
const [word, setWord] = useState(w);
const [isShow, setIsShow] = useState(false);
const [isDone, setIsDone] = useState(word.isDone);
@@ -9,7 +10,36 @@ export default function Word({ word }) {
}
//수정
function toggleDone() {
fetch(`http://localhost:3001/words/${word.id}`, {
method: "PUT",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
...word,
isDone: !isDone,
}),
}).then(res => {
if (res.ok) {
setIsDone(!isDone);
}
});
}
//삭제
function del() {
if (window.confirm("삭제 하시겠습니까?")) {
fetch(`http://localhost:3001/words/${word.id}`, {
method: "DELETE",
}).then(res => {
if (res.ok) {
setWord({ id: 0 });
}
});
}
}
if (word.id === 0) {
return null;
}
return (
@@ -21,8 +51,15 @@ export default function Word({ word }) {
<td>{isShow && word.kor}</td>
<td>
<button onClick={toggleShow}>뜻 {isShow ? "숨기기" : "보기"}</button>
<button onClick={del} className="btn_del">
삭제
</button>
</td>
</tr>
);
}
// Create - POST
// Read - GET
// Update - PUT
// Delete - DELETE